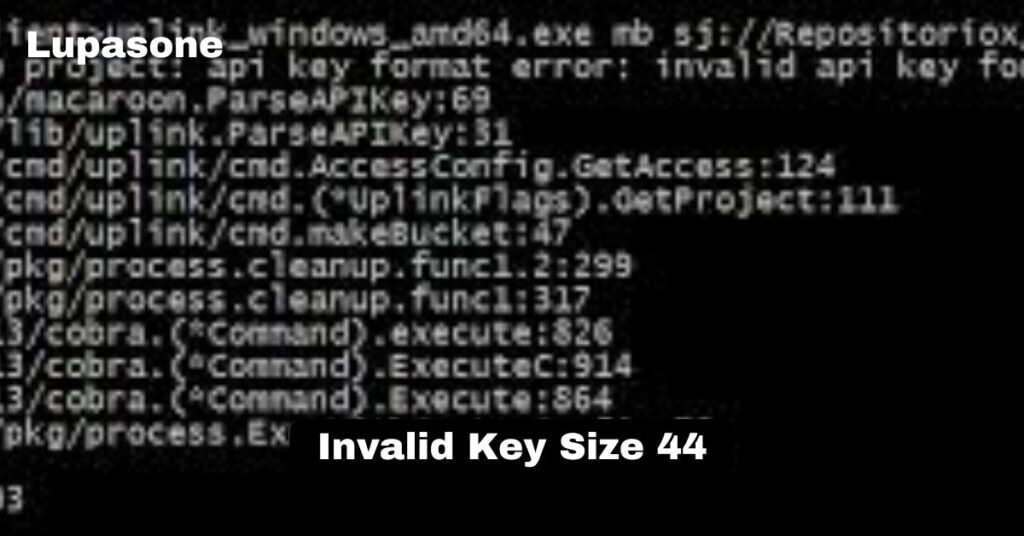
Contents
- 0.1 Overview of the AES Encryption Algorithm
- 0.2 Importance of Proper Key Sizes in AES Encryption
- 0.3 Introduction to the Key Size Error
- 0.4 What Does the “Panic: crypto/aes: Invalid Key Size 44 Error” Error Mean?
- 0.5 Why Key Size Matters in AES Encryption and What Could Trigger This Error
- 1 What Causes the Panic: crypto/aes: invalid key size 44 Error?
- 2 How to Fix the Panic: crypto/aes: invalid key size 44 Error
- 3 Best Practices for AES Encryption
- 4 Example Code Fixes for the Panic: crypto/aes: Invalid Key Size 44 Error
- 5 FAQs About Panic: crypto/aes: Invalid Key Size 44 Error
- 6 Conclusion: Avoiding the Panic: crypto/aes: Invalid Key Size 44 Error Error
- 7 Takeaway Points
Overview of the AES Encryption Algorithm
AES, or Advanced Encryption Standard, is a widely adopted symmetric-key encryption algorithm. It’s used to encrypt and decrypt data, ensuring confidentiality and security. AES is a cornerstone of modern cryptography, employed in various applications from secure communication to data storage.
Importance of Proper Key Sizes in AES Encryption
The strength of AES encryption hinges on the key size used. The key acts as a secret code, and its length determines the complexity of the encryption process. A longer key size translates to a more robust encryption, making it significantly harder for unauthorized parties to crack the code.
Introduction to the Key Size Error
The “Panic: crypto/aes: Invalid Key Size 44 Error ” error typically arises when an incorrect key size is used with the AES encryption algorithm. AES supports specific key sizes, and if a key that doesn’t conform to these standards is used, the error message appears. This indicates a misconfiguration or programming error in the system attempting to use AES.
What Does the “Panic: crypto/aes: Invalid Key Size 44 Error” Error Mean?
This error message specifically indicates that a key size of 44 bytes is being used with AES, which is an invalid size. A key size of 44 bytes (which is equivalent to 352 bits) falls outside these accepted ranges.
Why Key Size Matters in AES Encryption and What Could Trigger This Error
The key size in AES encryption is crucial for security. A larger key size increases the number of possible combinations for the key, making it exponentially more difficult for an attacker to brute force the encryption.
- Incorrect Key Generation: If the key is generated using an incorrect method or algorithm, it might result in an Invalid Key Size 44 Error.
- Data Corruption: Corrupted data can lead to unexpected values, including an Invalid Key Size 44 Error.
- Programming Errors: Mistakes in the code that handles AES encryption can cause incorrect key sizes to be used.
- Misconfiguration: Incorrect settings or configurations in the system or application using AES can result in Invalid Key Size 44 Error.
What Causes the Panic: crypto/aes: invalid key size 44 Error?
Overview of AES Key Sizes
AES supports three standard key sizes: 128-bit, 192-bit, and 256-bit. These key sizes determine the complexity of the encryption process and the level of security it provides. A 44-bit key size is not supported by AES, as it falls outside the accepted range.
Incorrect Key Handling in Code
Common mistakes in key management that can lead to the “panic: crypto/aes: Invalid Key Size 44 Error” error include:
- Incorrect input data length: If the input data length is not compatible with the chosen key size, it can result in an Invalid Key Size 44 Error.
- Malformed key input: Corrupted or malformed key data can also trigger the error.
- Bugs in the encryption code: Errors or inconsistencies in the encryption code can lead to incorrect key handling.
Misuse of Key Derivation Functions (KDFs)
Key derivation functions (KDFs) are used to generate encryption keys from passwords or other secret values. Misuse or misconfiguration of KDFs can result in improper key sizes. For example, if a KDF is used incorrectly to derive a key, it might produce a key that doesn’t conform to AES’s accepted sizes.
Mismatched Encryption Libraries
Using different encryption libraries or frameworks that interpret key sizes differently can also trigger this issue. If one library expects a key size in bytes, while another expects it in bits, it can lead to mismatches and errors.
How to Fix the Panic: crypto/aes: invalid key size 44 Error
Verifying Your Key Size
To ensure that your encryption key is the correct size for AES, you can use various tools and techniques:
- Print key size: Print the key size to the console or log to verify its value.
- Use debugging tools: Employ debugging tools to inspect the key size during runtime.
- Check cryptographic library documentation: Refer to the documentation of the cryptographic library you’re using for specific guidance on key size verification.
Proper Key Generation and Handling
- Use cryptographic libraries: Leverage cryptographic libraries like OpenSSL, Crypto++, or Java’s JCE to generate secure and correctly sized AES keys. These libraries provide built-in functions for key generation and verification.
- Follow best practices: Adhere to best practices for key generation, such as using strong random number generators and avoiding hardcoding keys.
Using Key Derivation Functions Correctly
- Configure KDFs appropriately: Set the desired key length and iteration count in your KDF configuration to ensure that the derived key matches the AES standard sizes.
- Use recommended parameters: Refer to cryptographic best practices and recommendations for KDF parameters to avoid security vulnerabilities.
Ensuring Compatibility Between Cryptographic Libraries
- Check library compatibility: Verify that the cryptographic libraries you’re using are compatible and interpret key sizes consistently.
- Use trusted libraries: Opt for well-established and widely used cryptographic libraries to minimize the risk of compatibility issues.
- Consult documentation: Refer to the documentation of the libraries you’re using for specific compatibility information and guidance.
Best Practices for AES Encryption
Why Proper Key Size Matters in AES
The key size in AES encryption is a critical factor in determining its security and performance. Using an incorrect key size can have significant implications:
- Reduced Security: A smaller key size makes it easier for attackers to brute force the encryption, compromising the security of your data.
- Inefficient Performance: Incorrect key sizes can lead to unexpected behavior or performance issues in the encryption process.
Secure Key Storage and Management
- Use secure key management systems: Employ dedicated key management systems to store and manage encryption keys securely. These systems often incorporate advanced security measures like encryption, access controls, and auditing.
- Consider hardware security modules (HSMs): HSMs provide a hardware-based solution for storing and managing cryptographic keys, offering a high level of security against unauthorized access.
Common Mistakes to Avoid When Using AES
- Incorrect key generation: Avoid generating keys using insecure methods or hardcoding them directly into your code.
- Misuse of key derivation functions: Ensure that KDFs are configured correctly to generate keys of the appropriate size.
- Inconsistent key usage: Use the same encryption key consistently throughout your application to avoid mismatches.
- Weak key storage: Avoid storing keys in plain text or in easily accessible locations.
- Incorrect padding: Ensure that the data being encrypted is padded correctly to align with the block size of the AES algorithm.
- Library vulnerabilities: Stay updated with the latest security patches for the cryptographic libraries you’re using to address known vulnerabilities.
Example Code Fixes for the Panic: crypto/aes: Invalid Key Size 44 Error
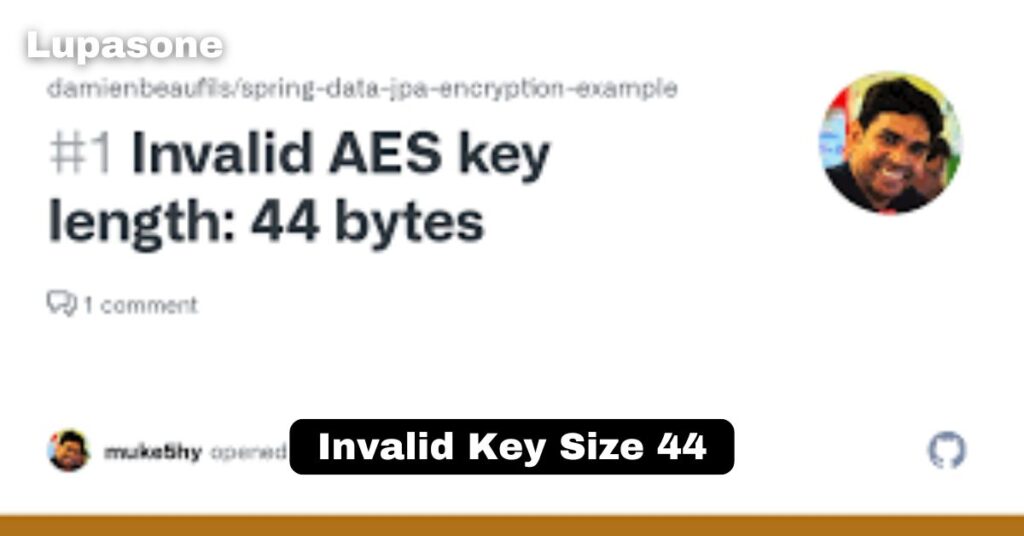
Example 1 – Incorrect Key Size Input
Go
// Incorrect key size input
key := []byte(“abcdefghijklmnopqrstuvwxyz”) // 26 bytes (208 bits)
// … encryption code using key …
Use code with caution.
To fix this, verify the key size before using it:
Go
if len(key) != 16 && len(key) != 24 && len(key) != 32 {
panic(“Invalid key size”)
}
// … encryption code using key …
Use code with caution.
Example 2 – Proper Use of KDF for AES Key Generation
Go
package main
import (
“crypto/aes”
“crypto/rand”
“golang.org/x/crypto/pbkdf2”
)
func main() {
// Generate a random salt
salt := make([]byte, 16)
_, err := rand.Read(salt)
if err != nil {
panic(err)
}
// Derive a 256-bit AES key using PBKDF2
key := pbkdf2.Key([]byte(“your_password”), salt, 10000, 32, aes.BlockSize)
// Use the derived key for encryption
// … encryption code using key …
}
Use code with caution.
Example 3 – Handling Library Compatibility Issues
Go
import (
“crypto/aes”
“crypto/cipher”
“crypto/rand”
)
func main() {
// Generate a random 256-bit key
key := make([]byte, 32)
_, err := rand.Read(key)
if err != nil {
panic(err)
}
// Create an AES cipher using the correct key size
block, err := aes.NewCipher(key)
if err != nil {
panic(err)
}
// … encryption code using block and key …
}
Use code with caution.
In this example, we use the crypto/aes package from the Go standard library to ensure compatibility and avoid key size errors.
FAQs About Panic: crypto/aes: Invalid Key Size 44 Error
What are the valid key sizes for AES encryption?
AES supports three standard key sizes: 128-bit, 192-bit, and 256-bit. These key sizes determine the strength and complexity of the encryption.
Why am I getting the “Invalid Key Size 44 Error” error?
You’re getting this error because you’re attempting to use an invalid key size with the AES encryption algorithm. AES only accepts the three standard key sizes mentioned above.
How do I generate a valid AES key?
You can generate a valid AES key using cryptographic libraries like OpenSSL, Crypto++, or Java’s JCE. These libraries provide functions for generating random keys of the correct size. Alternatively, you can use key derivation functions (KDFs) to derive a key from a password or passphrase.
Can I use a custom key size with AES encryption?
No, you cannot use a custom key size with AES encryption. The algorithm is designed to work with the specific key sizes of 128, 192, and 256 bits.
Which cryptographic libraries are recommended for AES encryption?
Several cryptographic libraries are well-regarded for AES encryption, including OpenSSL, Crypto++, Bouncy Castle, and the Java Cryptography Architecture (JCA). These libraries offer robust implementations of AES and provide various features for key management, encryption, and decryption.
Conclusion: Avoiding the Panic: crypto/aes: Invalid Key Size 44 Error Error
The “panic: crypto/aes: invalid key size 44” error can be a frustrating and potentially security-critical issue. By understanding the underlying causes and following best practices, you can effectively prevent this error and ensure the security of your AES encryption.
Summary of Key Points
- Valid key sizes: AES supports only 128-bit, 192-bit, and 256-bit key sizes.
- Incorrect key handling: Common mistakes include using incorrect key sizes, misusing KDFs, or encountering library compatibility issues.
- Best practices: Generate keys securely, store them properly, and use cryptographic libraries correctly.
- Error prevention: Verify key sizes, follow best practices for key management, and stay updated with the latest security recommendations.
Final Thoughts
By implementing secure and correct AES encryption, you can protect your data from unauthorized access. Staying informed about cryptographic best practices and addressing common mistakes like the “panic: crypto/aes: invalid key size 44” error will help you maintain a strong security posture.
Takeaway Points
- AES encryption only supports specific key sizes: Ensure that your keys are 128, 192, or 256 bits long.
- The “invalid key size 44” error is caused by improper key size input: Verify that you’re using the correct key size and handling it correctly.
- Properly configured key derivation functions (KDFs) and compatible libraries are essential: Use KDFs to generate secure keys and ensure that your cryptographic libraries work together seamlessly.
- Following encryption best practices ensures secure and error-free implementation: Adhere to recommended guidelines for key generation, storage, and usage to avoid common mistakes.
Visit home fo more articles